项目背景
- vue 2.6
- vue-class-component 7.2
- vuex 3.4
- vuex-class 3.4
- vue-router 3.2
- element-ui 2.14
- vue-property-decorator 8.4
- vue-cli 4
- webpack 4
今天抽空做一下 webpack 的打包优化,然后网上看看各种方式,一种是多进程打包
HappyPack
,项目使用TypeScript
尝试了下,由于vue-cli
配置有限,尝试几次失败后,选择简单的动态库的形式。
按照教程慢慢来
先写个 dll
的配置
webpack.dll.config.js
1 | // 标准的配置,没有什么异常 |
然后 添加 npm scripts
: "dll": "webpack --config ./webpack.dll.config.js"`
再修改 vue.config.js
这里需要安装一些依赖
npm i -d webpack-cli add-asset-html-webpack-plugin
随带添加了个 代码包分析插件 webpack-bundle-analyzer
, 打包速度分析插件 speed-measure-webpack-plugin
1 | // vue.config.js 部分内容 |
然后打包发布
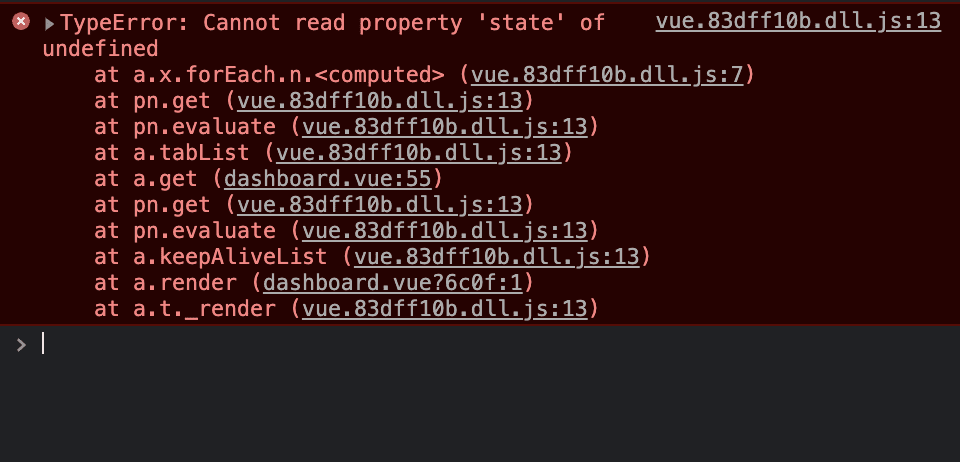
pa 哦豁,报错了,然后看到是在 vue.**.dll.js
报的错。
改下配置,将 vue 全家桶拆分
1 | entry: { |
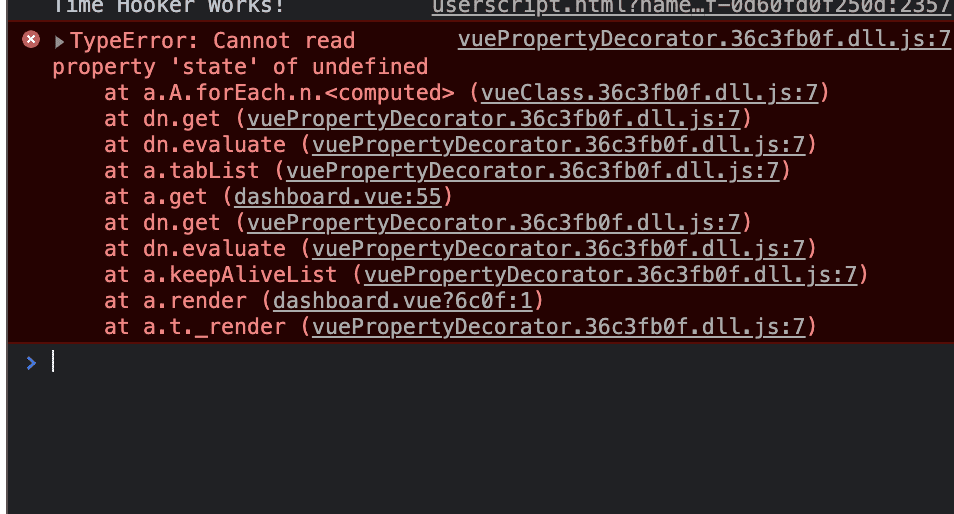
还是有问题,不过这次定位到了在 vue-property-decorator
里面,
修改配置,去掉 vue-property-decorator
1 | // 修改配置,去掉vue-property-decorator |
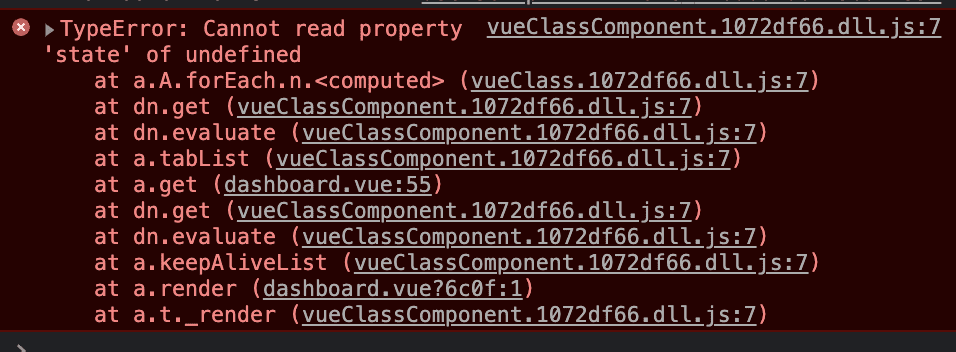
shit 再去掉 vue-class-component
1 | // 修改配置,再去掉vue-class-component |
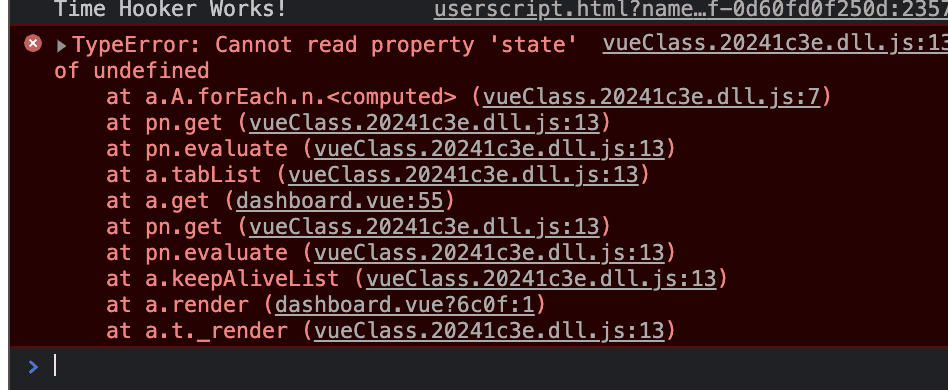
fuck 再去
1 | // 修改配置,再去掉vue-class-component |
nice 一切(看越来)正常
OMG, vue 怎么还在
vue
明明是已经抽取 dll
了,为什么打包的时候还有。。。
等我排查排查
此处省略 1W+字 😩😩😩
最后的配置
1 | entry: { |
perfect
你以为这就结束了?no no no
看一下打包的 dll
element.dll.js
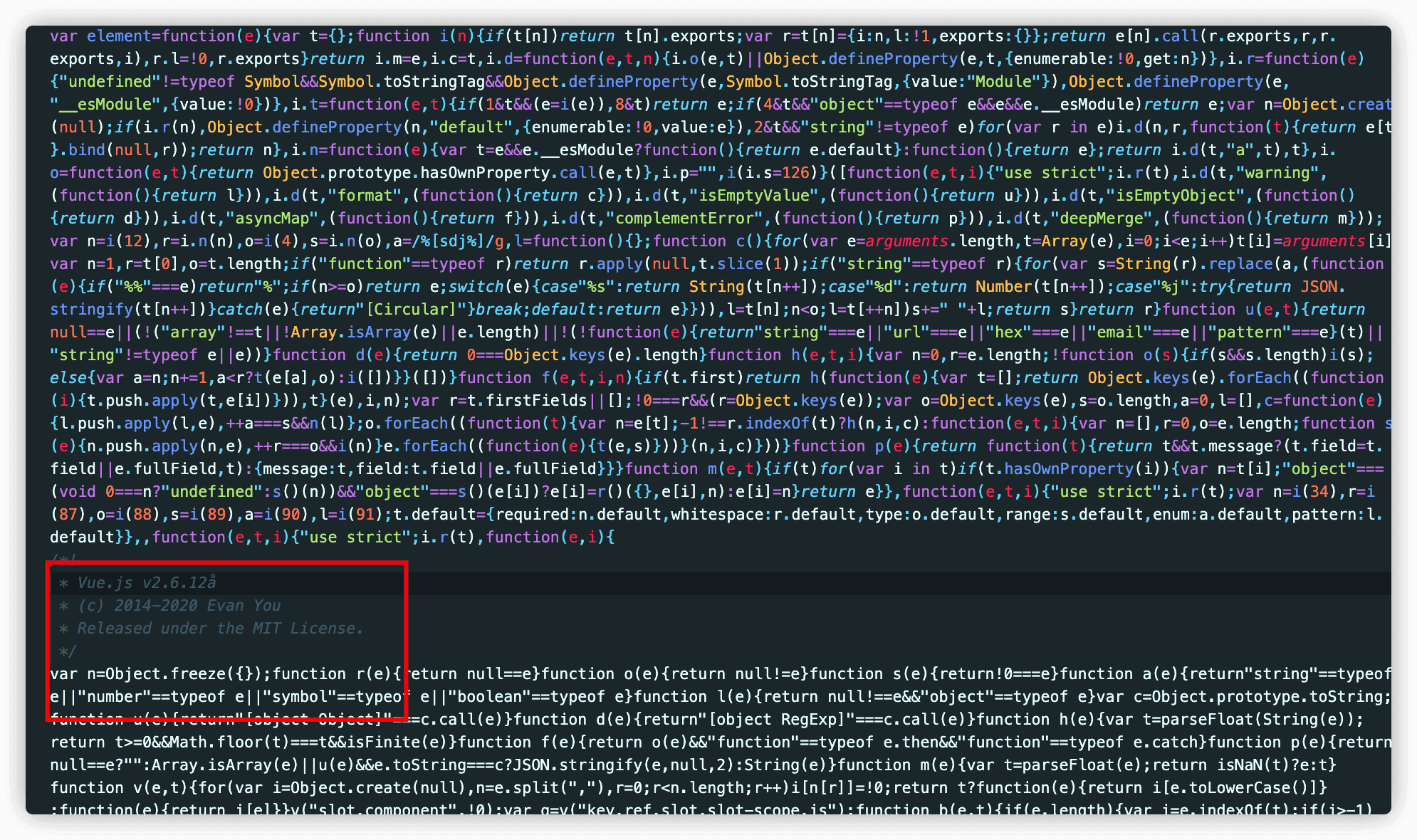
瞎搞,你element
怎么打vue
也打包了
再看看vue.dll.jd
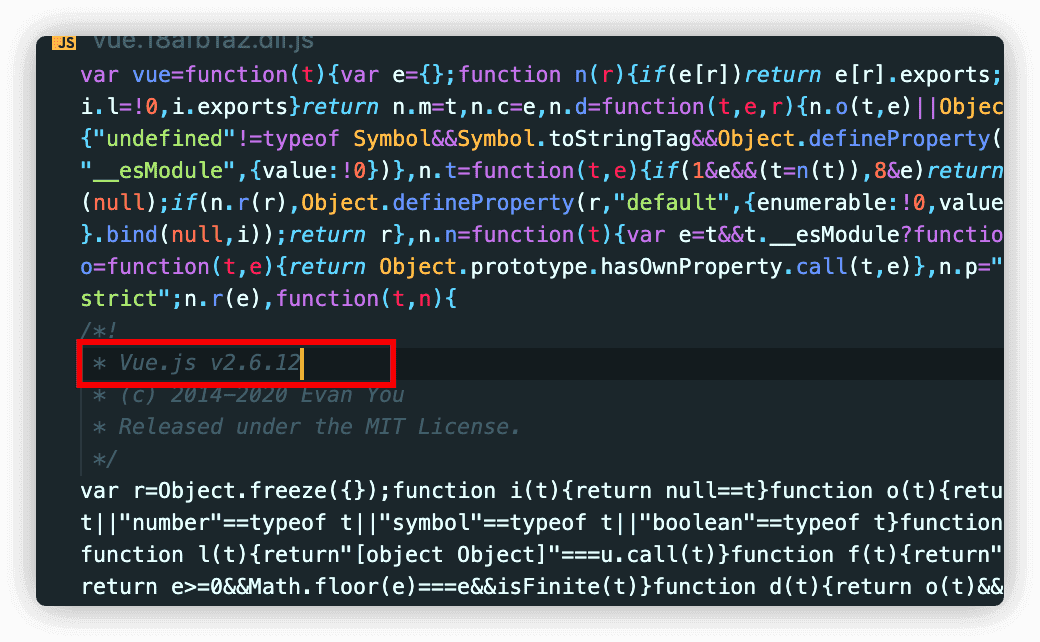
正常的,我自己打包自己没毛病吧
1 | 继续修改配置, |
把element-ui
和vue
一起打包,但是还是会打包多个,这里就不贴图了
继续翻翻翻翻 全局搜索的时候发现了这个
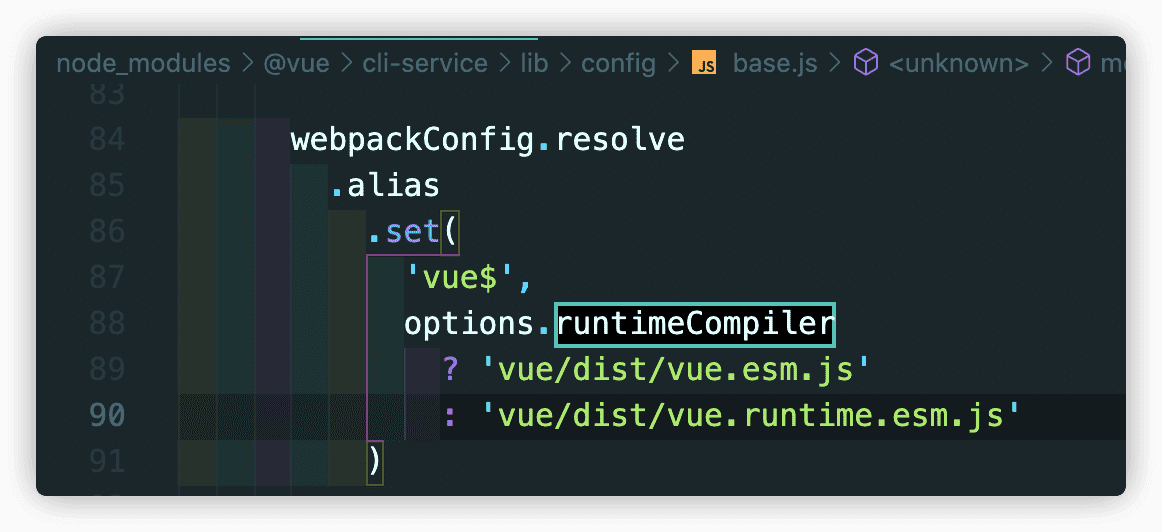
然后再看看 vue.config.js
1 | runtimeCompiler: true |
原来在这,改成false
就行了
OK 一切正常,这次是真 OK 了
附上最后的配置
1 | // 切记vue.config.js 配置 |
总结
一切的一切全来源于runtimeCompiler: true
成果
这是优化前的编译时间

这是优化后的编译时间

卧槽卧槽卧槽。。提速 95%...